How to Enhance Your Trading Skills
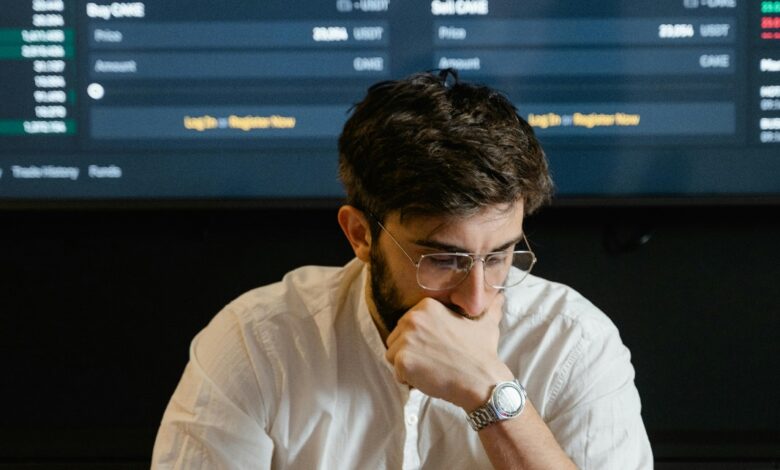
Do you want your trading game to improve? By following simple guidelines, you can enhance your abilities and boost your trading performance.
Here are some effective ways to enhance your trading skills.
Understand Market Trends and Trading Strategies
You must grasp the market thoroughly if you want to improve your trading skills. What’s very important is learning about many trading techniques. While some traders trade fast, others hang onto stocks for a lengthy period. Knowing what fits your style best will guide your decisions. Plainly put, having a strong foundation of market knowledge and various strategies helps you become a more skillful trader. Luckily, prop trading firms can help enhance your trading, as you have opportunities to hone your strategies and better grasp market trends.
Make a Solid Trading Plan
For success in trading, one needs a well-made plan. This strategy guides you through the tricky aspects of trading. Your step-by-step guide outlines when to buy and sell assets, preventing hasty decisions influenced by fleeting feelings. A good plan includes your investment goals, mind tolerance, and methods to follow. Sticking to the plan becomes key, especially in times of market fluctuation. Surges and dips may confuse many traders, but a well-structured guide helps you stay focused and act logically. Imagine trading without a plan as driving blindfolded—quite risky! So, for effective and consistent trading, always draft and adhere to a sound strategy.
Review Your Trades Regularly
Consistently examining your trades helps you spot strengths and weaknesses. This repeated practice gives insight into what methods pay off and which ones fall short. When you take time to study, it lets you understand if you’re sticking to your trading plan or if emotions are swaying your decisions. It becomes even more vital during periods of loss, as reviewing helps adapt strategies to counter the downturn. Many seasoned traders advocate maintaining a daily log of trades, listing their reasons for each action taken and noting the outcome. Such regular checks shape improved decision-making skills, thus advancing your overall trading competence.
Control Your Emotions
Controlling feelings is important for good trading. Sometimes, traders make snap decisions based on feelings like joy or despair; this can lead to losses. When the market is doing well, remain cool and avoid becoming too happy; when it declines, avoid panicking. Being even-tempered enables you to behave based on your trading strategy rather than out of impulse. Keeping your cool makes you a better trader.
Conclusion
Enhancing your trading skills takes effort and thoughtful methods. Overall, good trading relies on practice; your development journey through these suggestions will surely lead to greater success.